How to catch a message
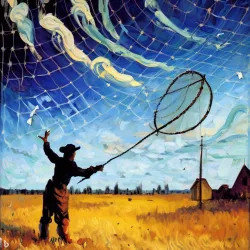
A message sent to a target can be handled by the target in a message handler. The message handler must be registered for this - preferably in the constructor of the target.
CMyTarget() { addMessageHandler(CRecordMyMessage.ID, this::asyncMyMessage); }
Here the message CRecordMyMessage
is handled by the handler asyncMyMessage
.
private boolean asyncMyMessage(@NotNull final CEnvelope aEnvelope, @NotNull final CRecord aRecord) { if (aEnvelope.isAnswer()) { // do something... return false; } else { // do something... aEnvelope.setResultSuccess(); return true; } }
Requests are processed as well as responses in a single handler. It is safer to always check the message for the response flag, even if a request or response is not expected.
In the case of requests, it is also important to set a result code.
If the requester checks the response, he should find the correct code.
If the code is not explicitly set by the recipient, the response contains the code NOT_HANDLED
, which
is not true in this case, since the recipient was able to receive the message.
If the message was handled, the handler is exited with true
.
Catch all remaining messages in one handler
It is possible to catch messages together in one handler that were not registered in the message handler registry of the target.
addMessageHandler(null, this::asyncNullHandler);