How to build a message
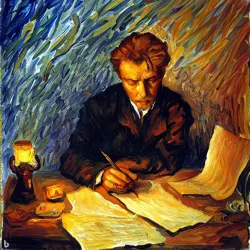
A message consists of an envelope and a record. The envelope contains data that controls the transport of the message to its recipient, similar to a letter. The record is the payload of the message. It consists of a map of keys and values. A key-value pair is called a slot. In addition, the record has an ID, also known as a message ID.
A cumbersome but working way of creating messages is to assemble them manually. The problem remains that the recipient must know exactly how the message is structured in order to access the content.
private void sendMessage(@NotNull final CTargetAddress aAddress) throws CException { final ISlotFactory slotFactory = CServiceRegistry.getInstance() .getServiceOrThrow(ISlotFactory.class); final CEnvelope env = CEnvelope.forSingleTarget(aAddress); final IId id = CIdFactory.fromObject("MyId"); final CRecord record = new CRecord(id); // integer ISlot slot = slotFactory.fromObject(CCommonSlotType.INT, 6); record.addSlot("key1", slot); // string slot = slotFactory.fromObject(CCommonSlotType.STRING, "Jim"); record.addSlot("key2", slot); // LocalDate slot = slotFactory.fromObject(CCommonSlotType.LOCAL_DATE, LocalDate.now()); record.addSlot("key3", slot); sendRequest(env, record); }
Defining Records in JSON
Much easier is the definition of records in JSON. From this JSON file, a class is generated with a supplied tool, the Record Generator, which can be used to write and read the data in records in a simple and type-safe manner.
The use of the generator is simple. It is a tool with a swing interface, which can remain open at all times during development. One click of a button is all it takes to generate record helper classes for all new or changed message definitions in a flash.
Okay, let's define the record from above in JSON.
{ "records": [ { "id": "MyId", "name": "MY_MESSAGE", "isService": "false", "namespaces": "", "description": "An example of a message defined in JSON", "slots": [ { "key": "key1", "name": "MY_INT", "direction": "REQUEST", "mandatory": "true", "type": "INT", "description": "An integer." }, { "key": "key2", "name": "MY_STRING", "direction": "REQUEST", "mandatory": "true", "type": "STRING", "description": "A string." }, { "key": "key3", "name": "MY_LOCAL_DATE", "direction": "REQUEST", "mandatory": "true", "type": "LOCAL_DATE", "description": "A local date." } ] } ] }
Okay, that's even more text. But with copy & paste record definitions are quickly created. In addition, you get an API that can be easily used in other projects. Later on also in projects for other languages than JAVA. And best of all, there is no need to pass interfaces or classes.
Now we still need to convert the record definition into an auxiliary class.
The record generator needs a root directory where it can search for record.json
or
record.xml
files.
In this directory (and all subdirectories) it creates the corresponding auxiliary classes, one for each record
definition in the files.
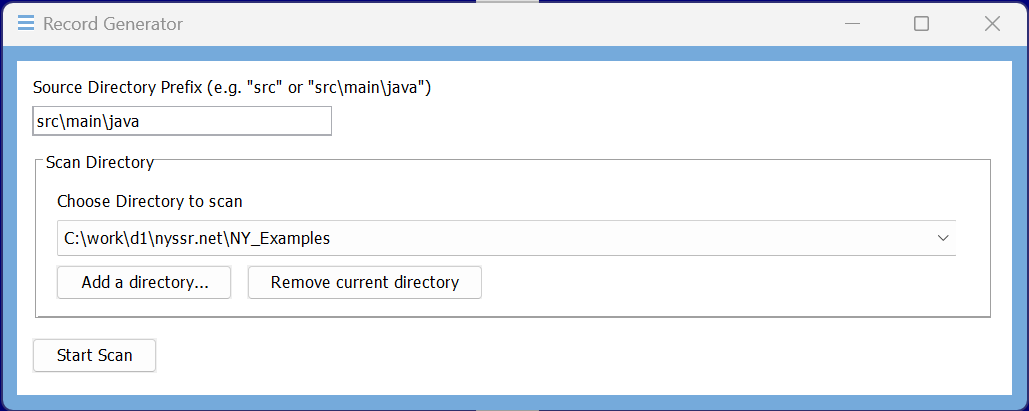
Let's build a message now with the auxiliary class.
private void sendMessage2(@NotNull final CTargetAddress aAddress) throws CException { final CEnvelope env = CEnvelope.forSingleTarget(aAddress); final CRecord record = CRecordMyMessage.create(); CRecordMyMessage.setMyInt(record, 6); CRecordMyMessage.setMyString(record, "Jim"); CRecordMyMessage.setMyLocalDate(record, LocalDate.now()); sendRequest(env, record); }