How to send a message
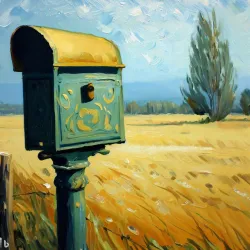
A message consists of an envelope and a record. The envelope contains data that controls the transport of the message to its recipient, similar to a letter. The record is the payload of the message. It consists of a map of keys and values. A key-value pair is called a slot. In addition, the record has an ID, also known as a message ID.
Sending a message to a known receiver
A message can be sent directly to a receiver if its target address is known. It is stored in the envelope, as is the sender's address.
public final void ping(@NotNull final CTargetAddress aReceiver) throws CException { final CEnvelope env = CEnvelope.forSingleTarget(aReceiver); env.setLogEnabled(false); final CRecord rec = new CRecord(CRecordPing.ID); CRecordPing.setLoop(rec, mPingCounter++); sendRequest(env, rec); }
Sending a message to a local nano service
A message can be sent directly to a nano service. Since nano services are bound to the namespace, you only need to specify the namespace in the envelope. The SYSTEM namespace is responsible for nano services that are in charge of the entire node. All observers who stick to the nano service get a copy of the message.
private void sendNotifyNodeRecordUpdated(@NotNull final CNodeAddress aRemoteNode) throws CException { final CEnvelope env = CEnvelope.forLocalNanoService(CWellKnownNID.SYSTEM); final CRecord rec = CRecordNetworkNotifyNodeRecordUpdated.create(); CRecordNetworkNotifyNodeRecordUpdated.setRemoteNode(rec, aRemoteNode); sendNotification(env, rec); }
Sending a message to a remote nano service
Sending a message to a nano service in another node is also a common action. In addition to the namespace, the node must also be specified in the envelope.
private void sendBroadCast(@NotNull final CMessage aMsgToSend, @NotNull final IId aNamespaceId, @NotNull final CNodeId aNodeId) { final CEnvelope env = CEnvelope.forRemoteNanoService(aNamespaceId, aNodeId); final CRecord rec = CRecordBroadcastSendMsg.create(); CRecordBroadcastSendMsg.setLocally(rec, true); CRecordBroadcastSendMsg.setMessage(rec, msgToSend); sendNotification(env, rec); }
Sending a Message to a micro service
To send a message to a MicroService, we only need the microservice ID. It does not matter whether the microservice is running on the same node or on a different node, and whether there are one or more microservices with this ID.
private static void sendValidateToken(@NotNull final CTarget aTarget, @Nullable final UUID aSessionToken) throws CException { final IId microServiceId = CIdFactory.fromObject("ccf168c1-f18b-4229-85f9-24461a19ee6a"); final CEnvelope env = CEnvelope.forMicroService(microServiceId); final CRecord record = CRecordSessionValidate.create(); CRecordSessionValidate.setToken(record, aSessionToken); aTarget.sendRequest(env, record); }
Sending a broadcast message
A broadcast message is a message intended for a nano service (usually in the SYSTEM namespace) in all nodes simultaneously. A nano service in the plugin NY_Network is responsible for sending broadcasts. The desired message is simply sent to this local nano service, which then sends the broadcast to all nodes. The record must also be a nano service, so that all targets that have attached themselves to this nano service as observers receive a copy of the message.
void broadCast(@NotNull final CEnvelope aEnvelope, @NotNull final CRecord aRecord) throws CException { aEnvelope.setSender(getAddress()); final CMessage msgToSend = new CMessage(aEnvelope, aRecord); final CEnvelope env = CEnvelope.forLocalNanoService(CWellKnownNID.SYSTEM); final CRecord rec = CRecordBroadcastSendMsg.create(); CRecordBroadcastSendMsg.setLocally(rec, true); CRecordBroadcastSendMsg.setMessage(rec, msgToSend); send(env, rec); }