The use of timer messages
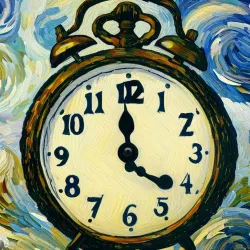
Timers in
As always, we assume that the package has been initialized. Here are the dependencies we have:
@Override public void getDependencies(@NotNull final IServiceDependencyList aDependencyList) { aDependencyList.add(INamespaceRegistry.class); aDependencyList.add(ITimerManager.class); }
We need the namespace registry to fetch a namespace in which we can register the target. For the timer we need the Timer Manager.
class CMyTarget extends CTarget implements IService { private static final IId MY_TIMER_ID = CIdFactory.fromObject("MyTimerId"); private static final long TIMEOUT = 1000L; private final IDependencies mDependencies; // can be used to stop or restart timer private long mTimerHandle = ITimerManager.INVALID; CMyTarget(@NotNull final IDependencies aDependencies) { mDependencies = aDependencies; addMessageHandler(CRecordStartTarget.ID, this::asyncStartTarget);// add message handler for timer addMessageHandler(MY_TIMER_ID, this::asyncTimer); } private boolean asyncStartTarget(@NotNull final CEnvelope aEnvelope, @NotNull final CRecord aRecord) { // In the SYSTEM namespace thread// start Timer mTimerHandle = mDependencies.getTimerManager() .createAndStartTimer(MY_TIMER_ID, getAddress(), TIMEOUT, false, null); aEnvelope.setResultSuccess(); return true; }// handle Timer private boolean asyncTimer(@NotNull final CEnvelope aEnvelope, @NotNull final CRecord aRecord) { // do something aEnvelope.setResultSuccess(); return true; } @Override public void activate(@NotNull final IServiceRegistry aServiceRegistry) throws Exception { final INamespace namespace = mDependencies.getNamespaceRegistry() .getNamespace(CWellKnownNID.SYSTEM); assert namespace != null; namespace.getTargetRegistry() .registerTarget(this); } @Override public void deactivate(@NotNull final IServiceRegistry aServiceRegistry) throws Exception { deregisterTarget(); } }