IJobEngine
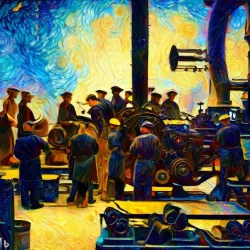
The Job Engine executes jobs in multiple threads simultaneously. It is created by the Job Engine factory.
Get a job engine
The job engine is available via the job engine factory. This in turn is a singleton, which is obtained via the service registry.
final IJobEngineFactory factory = CServiceRegistry.getInstance() .getService(IJobEngineFactory.class); final IJobEngine engine = factory.createEngine("MyEngine");
As always, the package starter should have a dependency on the job engine factory to make sure it is already initialized.
Adding threads
The Job Engine requires at least one thread to execute. We can simply add threads with a method.
void appendThread(@NotNull String aName, @NotNull EThreadPriority aPriority, int aMaxConcurrentJobs) throws CException;
engine.appendThread("MyEngine_1", EThreadPriority.NORMAL, 10); engine.appendThread("MyEngine_2", EThreadPriority.NORMAL, 10); engine.appendThread("MyEngine_3", EThreadPriority.NORMAL, 10);
Setting the owner
You can assign an owner to the job machine. The owner will be notified by a message (CRecordNoMoreJobs) as soon as all jobs have been completed.
engine.setOwner(getAddress());
Add a message handler in the constructor of your target.
addMessageHandler(CRecordNoMoreJobs.ID, this::asyncNoMoreJobs);
Your message handler looks like:
private boolean asyncNoMoreJobs(@NotNull final CEnvelope aEnvelope, @NotNull final CRecord aRecord) { if (aEnvelope.isAnswer()) { return false; } else { // ... return true; } }
Add jobs to the engine
Jobs are transferred internally asynchronously to the machine via message. If enough jobs are already running, the new job will wait in a queue to be deployed.
void appendJob(@NotNull IJob aJob);
Start the job engine
void start() throws CException;
Remove a job from the engine
Removes a job from the job engine. It does not matter whether the job is still waiting to be started or has already been started.
void removeJob(@NotNull IJob aJob);
Getter
Get the number of all jobs currently running.
int getRunningJobCount();
Get the number of all jobs waiting to be executed.
int getWaitingJobCount();
Dismiss
Dismiss the job engine.
void dismiss() throws CException;